React development is actually one of the most popular interface creation libraries. Developed by Facebook, it boasts a set of features that let you easily build fast, dynamic, and scalable web applications using minimal effort. If you just started programming, then React definitely will be a great choice to build modern performance web applications.
Advantages of Using React
- Easy to learn: React is straightforward to pick up, especially if you already know JavaScript. Its concepts are simple, and it has a huge and crystal-clear documentation.
- Component-based approach: Breaking down the application into smaller components helps to declutter the code and makes it easier to read. Every component in React represents an independent unit, making testing and support much easier.
- VirtualDOM: React maintains a virtual DOM, which improves page refresh speeds. On a change in data, it first updates the virtual DOM and then only those real elements on the page, which enormously speeds up an application’s work.
- Flexibility: Not only is React not limited to web applications, but with the help of React Native, you can also build mobile applications. This means you can use the same skills for development on different platforms.
- Large community: It’s not a library; React is a big ecosystem with several tools and libraries that are going to help you. You will find a large community of developers willing to help.
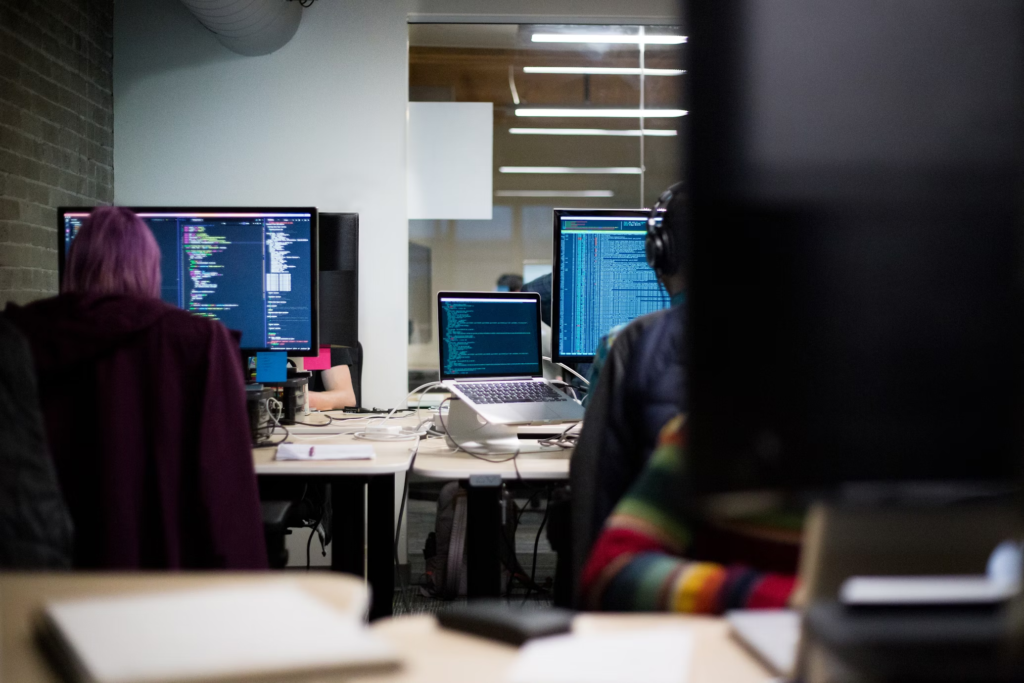
1. Setting up the Working Environment
Before we start working with React development, it will be better if you set up your working environment. It’s really simple, and Celadonsoft will show you through every little step so that you get into coding straight away.
1) Installation of Node.js and npm
The definition from the MVP development services team of Celadonsoft: “React requires an installation of Node.js, a JavaScript environment; the latter comes with npm, which will help you manage the dependencies and libraries needed for your project. To install Node.js, go to the official website and download the latest version of Long Term Support.” After installation, please check that everything went well by running the following command in the terminal:
node -v
npm -v
These commands will show the versions of Node.js and npm installed.
2) Creating a New Project Using Create React App
The easiest way to begin working with React development is by using the tool called Create React App. Immediately, it will configure your project using all the necessary dependencies and configurations:
npm create-react-app my-app
Rename “my-app” to the name of your project. After this command executes, it will create a folder containing the project and install all necessary libraries. When the installation is complete, go to the project directory:
cd my-app
Now, to check that everything is working properly, launch the development server:
npm start
Then your browser will open the example page of a React-application.
3) Setting up Code Editor for Working with React
For comfort during the development process, we recommend using the Visual Studio Code editor. Among the developers it is one of the most popular editors, and for working with React it’s perfect.
With the VS Code setup done, put in some useful extensions: ESLint to lint your syntax errors and Prettier to autoformat code. These tools help maintain your code in a clean and correct state.
2. Basics of React: Components and JSX
React is a library that will help you build interfaces by breaking them into small chunks called components architecture. This comes in handy, especially because each component has its part of the interface and can be reused.
What Are Components?
A component, in the world of React, is basically a function or a class which returns HTML markup—or something that looks like HTML. For example, button, form, menu—all these can be components. Using components, you are able to build your interface — just as a designer — adding and combining as needed.
JSX Introduction
JSX is an extension to the syntax of the JavaScript language, allowing one to describe HTML-like constructs inside JavaScript. It increases the readability of a code and eases its maintenance.
JSX is not needed for React, but it makes the job much easier. It is important to remember that under the hood, JSX gets converted to JavaScript functions, so you can always use regular JavaScript if you want.
Pass Data Between Components Using Props
Sometimes components need to share data with each other. In React, this is achieved through props, short for “properties”. It’s a way of passing information from one component to another, much like parameters in functions.
The Greeting component accepts a prop called name and uses that to display a greeting personalized to the user. The components are hence flexible and reusable.
Component State Management
When developing web applications with React, it is critical to understand how to manage the state of components. State allows your components to store and update data without having to rewrite the entire page. In this section we will cover the basic principles of working with state, learn how to process events and deal with dynamic data rendering.
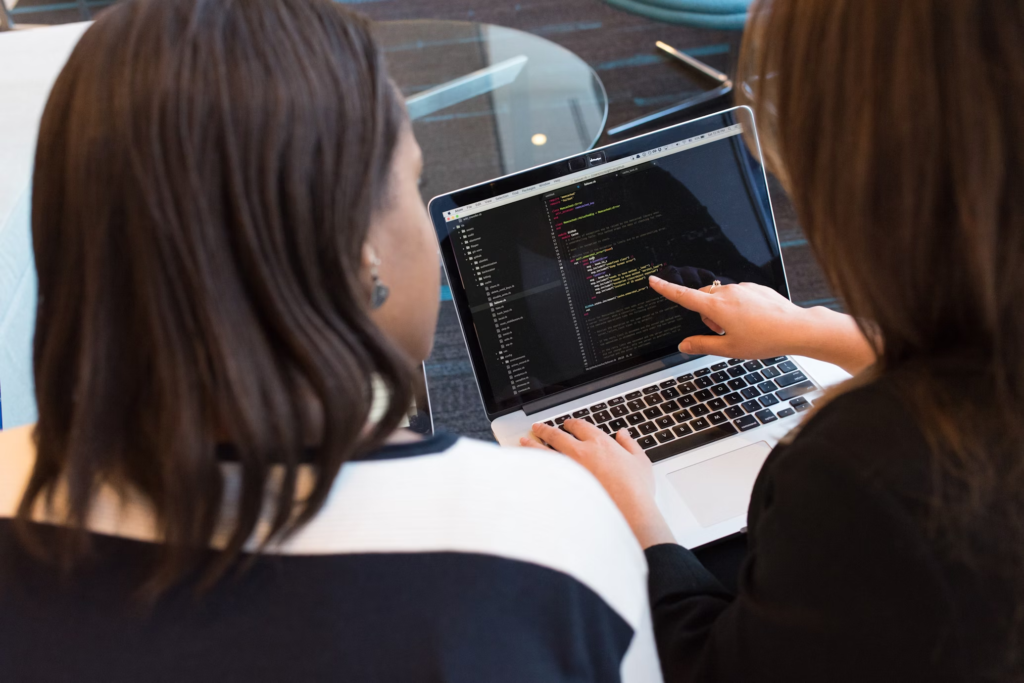
3. Management of the Component State
Each component in React can have its own state — it is a changeable data that affects how the user interface appears. For example, imagine the «Like» button that changes the text when you press it:
import { useState } from "react";
function LikeButton() {
const [liked, setLiked] = useState(false);
return (
<button onClick={() => setLiked(!liked)}>
{liked? " Like" : "Tab Like"}
</button>
);
}
export default LikeButton;
Here, we use the hooks as useState, which creates a liked variable and a setLiked function to modify it.
How Does Usestate Work?
The useState hook takes the initial value and returns an array of two elements:
- Current status value
- Function for its update
Each time setCount is called, React updates the component and displays the current value.
Event Handling and Status Update
In React event handlers work similarly to normal JavaScript, but with some differences:
- Use camelCase attributes (onClick, onChange instead of onclick, onchange)
- Processors are passed as functions, not strings
List Rendering and Conditional Rendering
React allows you to dynamically display lists of elements using the map() method.
4. Effective Interaction with API
In modern web applications, not only is an ability to work with the interface required, but a way to deliver data from the server is also to be implemented. This section reviews how React’s built-in capabilities make it easy and convenient for you to deal with the API.
Get Data from Server Using UseEffect
This approach allows you to load data immediately after mounting the component, but it is also indispensable for us to handle possible errors.
Asynchronous Requests and Errors Handling
When working with the server, various errors may occur: API unavailability, incorrect response or data loading delays. Let’s finish our code by adding the boot status and error handling:
function UsersList() {
useEffect(() => {
fetch("https://jsonplaceholder.typicode.com/users")
.then((response) => {
if (!response.ok) {
throw new Error("Error in the data loading");
}
return response.json();
})
.then((data) => {
setUsers(data);
setLoading(false);
})
.catch((error) => {
setError(error.message);
setLoading(false);
});
}, []);
if (loading) return <p>Loading...</p>;
if (error) return <p>Error: {error}</p>;
return (
<ul>
{users.map((user) => (
<li key={user.id}>{user.name}</li>
))}
</ul>
);
}
Now the component shows the user that data is being downloaded and if an error occurs, it displays a corresponding message.
Implementation of Paining and Filtering
In real applications, you often need to load data in parts, for example, not show the entire list of users at once, but 10 records at a time. It’s called pagination.
The simplest way to implement pagination is to download only the necessary data from the server, passing parameters in URL.
That’s much the same with filtering: instead of passing the page number, you can send other search parameters as query parameters.
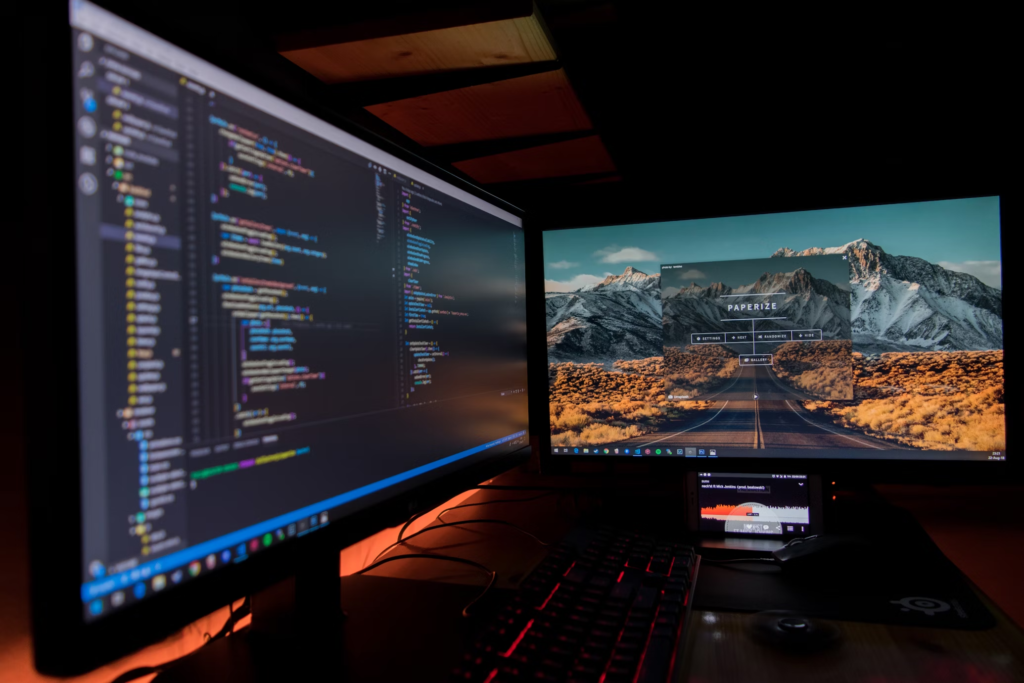
5. State Management with Redux
As your application becomes larger and more complex, managing the state through useState can become cumbersome. In such cases, Redux comes to the rescue — a powerful tool for working with the global state of the application.
Redux is built around three key concepts:
- Store is a centralized data storage. It stores all the status of your application.
- Actions are objects that describe what changes should be made to the data.
- Reducers — functions that accept the current state and action and return a new state.
6. React-application Testing
Writing tests may seem complicated, but it helps to avoid mistakes that can occur during the development process. In React, the most popular tools for testing are Jest and React Testing Library.
7. Application Deployment and Optimization
After you have developed your React application, it needs to be optimized and deployed so that it works quickly and smoothly in the production.
8. Routing in React: Creating Single-Page Applications (SPA)
Modern web applications often use the concept of SPA. In such applications, there is no traditional page restart when switching between sections. Instead, content is dynamically updated and navigation mimics the classic behavior of multipage sites.
React uses the React Router library most frequently to create an SPA. It allows you to organize routing, manage URLs and display the required components based on address.
Summing Up
We advise not to stop at theory, but immediately apply the knowledge in practice. Try to create a small project, experiment with different tools and approaches. If you have any questions, you can always contact the documentation or the developer community.